What is upcasting? Get up cast, or upcasting, is a term used in computer science and programming to refer to a scenario where a variable of a certain data type is converted to a variable of a higher data type. This is often done to take advantage of the larger range or precision of the higher data type, or to allow the variable to be used in a context where the higher data type is required.
For example, if you have a variable of type `int` (32-bit integer) and you want to store a value that is larger than the maximum value that can be represented by an `int`, you can upcast the variable to a `long` (64-bit integer) variable.
Upcasting is also used to allow objects of different classes to be treated as objects of a common superclass. This can be useful for writing generic code that can operate on objects of different types.
- Discover Marc Lamont Hills Wife Behind The Scenes Of The Renowned Intellectual
- Sheila Ebanas Birth A Comprehensive Examination
Upcasting is a powerful tool that can be used to improve the performance and flexibility of your code. However, it is important to use upcasting carefully, as it can also lead to errors if it is not used correctly.
Key Aspects of Upcasting
There are several key aspects of upcasting that are important to understand:
- Data loss: When you upcast a variable, you may lose precision or range. For example, if you upcast a `float` variable to a `double` variable, you may lose some of the precision of the `float` variable.
- Type safety: Upcasting can lead to type safety errors if it is not used correctly. For example, if you try to upcast a `String` variable to an `int` variable, you will get a type safety error.
- Performance: Upcasting can have a negative impact on performance, as it requires the compiler to generate additional code to handle the upcasting.
Benefits of Upcasting
Despite the potential drawbacks, upcasting can also provide several benefits:
- Ultimate Guide To Kirby Essential Tips And Tricks
- Meet Ha Ji Wons Husband Everything You Need To Know
- Increased flexibility: Upcasting allows you to write code that can operate on objects of different types. This can make your code more flexible and easier to maintain.
- Improved performance: Upcasting can improve the performance of your code by reducing the number of type conversions that are required.
- Reduced code complexity: Upcasting can help to reduce the complexity of your code by eliminating the need for conditional statements to handle different types of objects.
Conclusion
Upcasting is a powerful tool that can be used to improve the performance and flexibility of your code. However, it is important to use upcasting carefully, as it can also lead to errors if it is not used correctly.
Get up cast, also known as upcasting, is a programming technique that involves converting a variable of a certain data type to a variable of a higher data type. This is often done to take advantage of the larger range or precision of the higher data type, or to allow the variable to be used in a context where the higher data type is required.
- Data Loss: When you upcast a variable, you may lose precision or range.
- Type Safety: Upcasting can lead to type safety errors if it is not used correctly.
- Performance: Upcasting can have a negative impact on performance, as it requires the compiler to generate additional code to handle the upcasting.
- Increased Flexibility: Upcasting allows you to write code that can operate on objects of different types.
- Improved Performance: Upcasting can improve the performance of your code by reducing the number of type conversions that are required.
- Reduced Code Complexity: Upcasting can help to reduce the complexity of your code by eliminating the need for conditional statements to handle different types of objects.
- Code Reusability: Upcasting can help to improve code reusability by allowing you to write code that can be used with different types of objects.
- Improved Maintainability: Upcasting can help to improve the maintainability of your code by reducing the number of type-specific code that you need to write.
Upcasting is a powerful tool that can be used to improve the performance, flexibility, and maintainability of your code. However, it is important to use upcasting carefully, as it can also lead to errors if it is not used correctly.
Data Loss
Data loss is a potential issue that can occur when you upcast a variable. Upcasting is the process of converting a variable of a certain data type to a variable of a higher data type. This is often done to take advantage of the larger range or precision of the higher data type, or to allow the variable to be used in a context where the higher data type is required.
However, upcasting can also lead to data loss if the higher data type has a smaller range or precision than the original data type. For example, if you upcast a 32-bit integer to a 16-bit integer, you may lose some of the precision of the original value.
Data loss can also occur if the higher data type has a different range of values than the original data type. For example, if you upcast a signed 32-bit integer to an unsigned 32-bit integer, you may lose the ability to represent negative values.
It is important to be aware of the potential for data loss when upcasting variables. If data loss is a concern, you should consider using a different data type or using a casting method that does not result in data loss.
Here is an example of how data loss can occur when upcasting a variable:
int main() { int x = 1000000000; short y = (short) x; printf("x = %d, y = %d\n", x, y); return 0;}In this example, the variable `x` is a 32-bit integer with the value 1000000000. The variable `y` is a 16-bit integer. When the value of `x` is upcast to `y`, the precision of the value is lost and the value of `y` becomes -2147483648.
This example illustrates the importance of being aware of the potential for data loss when upcasting variables.
Type Safety
Type safety is a programming language feature that helps to prevent errors by ensuring that variables are used according to their declared type. Upcasting is a type of type conversion that can lead to type safety errors if it is not used correctly. This is because upcasting can allow a variable to be used in a context where it is not of the correct type.
For example, consider the following code:
int x = 10; Object y = x; y ="Hello"; x = (int) y;In this example, the variable `x` is declared as an `int`, and the variable `y` is declared as an `Object`. The value of `x` is then upcast to `y`. This is a valid operation because `int` is a subclass of `Object`. However, the next line of code assigns the value `"Hello"` to `y`. This is a type safety error because `y` is declared as an `Object`, and `Object` cannot store a value of type `String`. The final line of code attempts to downcast the value of `y` back to an `int`. This is also a type safety error because `y` now contains a value of type `String`, and `String` cannot be converted to an `int`.
Type safety errors can be difficult to find and debug. This is because the compiler will not always detect type safety errors. For example, the compiler will not detect the type safety error in the above code because the upcast from `int` to `Object` is a valid operation. However, the runtime environment will detect the type safety error when the attempt is made to assign the value `"Hello"` to `y`.
It is important to use upcasting carefully to avoid type safety errors. Upcasting should only be used when it is necessary to allow a variable to be used in a context where it is not of the correct type.
Performance
Upcasting can have a negative impact on performance because it requires the compiler to generate additional code to handle the upcasting. This additional code can slow down the execution of your program, especially if you are upcasting frequently.
- Compiler Overhead: When you upcast a variable, the compiler must generate additional code to check the type of the variable and to convert the variable to the new type. This additional code can add overhead to your program, especially if you are upcasting frequently.
- Runtime Overhead: In some cases, upcasting can also add overhead to the runtime of your program. This is because the runtime environment may need to perform additional checks to ensure that the upcasted variable is used correctly.
Here are some tips for minimizing the performance impact of upcasting:
- Avoid upcasting if possible.
- Upcast only when necessary.
- Use the most specific type possible.
Increased Flexibility
Upcasting is a powerful tool that can be used to increase the flexibility of your code. By upcasting, you can write code that can operate on objects of different types. This can be useful in a variety of situations, such as when you are working with collections of objects or when you are writing generic code.
For example, consider a collection of shapes. The collection may contain a variety of shapes, such as circles, squares, and triangles. If you want to write code that can operate on all of the shapes in the collection, you can upcast the shapes to a common superclass, such as the Shape class. Once the shapes have been upcast, you can use the Shape class's methods to operate on all of the shapes in the collection, regardless of their specific type.
Upcasting can also be used to write generic code. Generic code is code that can be used with different types of data. For example, you could write a generic sorting algorithm that can be used to sort any type of data that implements the Comparable interface. By using upcasting, the sorting algorithm can be written in a way that is independent of the specific type of data that is being sorted.
Upcasting is a powerful tool that can be used to increase the flexibility and reusability of your code. However, it is important to use upcasting carefully, as it can also lead to errors if it is not used correctly.
Improved Performance
Upcasting can improve the performance of your code by reducing the number of type conversions that are required. This is because the compiler can generate more efficient code when it knows the exact type of a variable. For example, if you have a variable of type `int`, the compiler can generate more efficient code than if you have a variable of type `Object`. This is because the compiler knows that an `int` variable can only hold a value between -2,147,483,648 and 2,147,483,647, while an `Object` variable can hold any type of value. As a result, the compiler can generate more efficient code for operations on `int` variables than for operations on `Object` variables.
- Reduced Number of Type Conversions: Upcasting reduces the number of type conversions that are required, which can improve the performance of your code.
- More Efficient Code Generation: The compiler can generate more efficient code when it knows the exact type of a variable, which can improve the performance of your code.
- Improved Performance for Primitive Types: Upcasting can improve the performance of your code for primitive types, such as `int` and `float`, because the compiler can generate more efficient code for these types.
Overall, upcasting can improve the performance of your code by reducing the number of type conversions that are required and by allowing the compiler to generate more efficient code.
Reduced Code Complexity
Upcasting is a powerful tool that can be used to reduce the complexity of your code. By upcasting, you can eliminate the need for conditional statements to handle different types of objects. This can make your code more readable, maintainable, and extensible.
For example, consider the following code:
if (object instanceof Circle) { Circle circle = (Circle) object; // Do something with the circle } else if (object instanceof Square) { Square square = (Square) object; // Do something with the square } else if (object instanceof Triangle) { Triangle triangle = (Triangle) object; // Do something with the triangle }This code uses conditional statements to handle different types of objects. This can make the code difficult to read, maintain, and extend. However, by upcasting the objects to a common superclass, such as the Shape class, you can eliminate the need for conditional statements. For example:
if (object instanceof Shape) { Shape shape = (Shape) object; // Do something with the shape }This code is much simpler and easier to read, maintain, and extend. Upcasting can also help to improve the performance of your code. By eliminating the need for conditional statements, you can reduce the number of branches in your code. This can make your code run faster and more efficiently.
Overall, upcasting is a powerful tool that can be used to reduce the complexity, improve the readability, and enhance the performance of your code. However, it is important to use upcasting carefully, as it can also lead to errors if it is not used correctly.
Code Reusability
Upcasting is a powerful technique that can be used to improve the reusability of your code. By upcasting, you can write code that can operate on objects of different types. This can be useful in a variety of situations, such as when you are working with collections of objects or when you are writing generic code.
For example, consider a collection of shapes. The collection may contain a variety of shapes, such as circles, squares, and triangles. If you want to write code that can operate on all of the shapes in the collection, you can upcast the shapes to a common superclass, such as the Shape class. Once the shapes have been upcast, you can use the Shape class's methods to operate on all of the shapes in the collection, regardless of their specific type.
Upcasting can also be used to write generic code. Generic code is code that can be used with different types of data. For example, you could write a generic sorting algorithm that can be used to sort any type of data that implements the Comparable interface. By using upcasting, the sorting algorithm can be written in a way that is independent of the specific type of data that is being sorted.
Overall, upcasting is a powerful tool that can be used to improve the reusability of your code. By upcasting, you can write code that can operate on objects of different types and that can be used in a variety of situations.
Here are some additional benefits of upcasting:
- Increased flexibility: Upcasting allows you to write code that is more flexible and can be used in a wider variety of situations.
- Improved maintainability: Upcasting can help to improve the maintainability of your code by reducing the number of conditional statements that are required to handle different types of objects.
- Reduced code duplication: Upcasting can help to reduce code duplication by allowing you to write code that can be reused in multiple situations.
Upcasting is a powerful tool that can be used to improve the reusability, flexibility, and maintainability of your code. However, it is important to use upcasting carefully, as it can also lead to errors if it is not used correctly.
Improved Maintainability
Upcasting is a powerful technique that can be used to improve the maintainability of your code. By upcasting, you can reduce the number of type-specific code that you need to write. This can make your code easier to read, understand, and maintain.
For example, consider the following code:
public class Shape { public void draw() { System.out.println("Drawing a shape..."); }}public class Circle extends Shape { public void draw() { System.out.println("Drawing a circle..."); }}public class Square extends Shape { public void draw() { System.out.println("Drawing a square..."); }}public class Main { public static void main(String[] args) { Shape shape1 = new Circle(); Shape shape2 = new Square(); shape1.draw(); shape2.draw(); }}
In this example, we have three classes: `Shape`, `Circle`, and `Square`. The `Shape` class is a superclass of the `Circle` and `Square` classes. The `draw()` method is defined in the `Shape` class and overridden in the `Circle` and `Square` classes.If we want to draw a circle or a square, we can create an instance of the `Circle` or `Square` class and call the `draw()` method on that instance. However, this code is not very maintainable because we have to write separate code for each type of shape that we want to draw.We can improve the maintainability of this code by using upcasting. Upcasting is the process of converting an object of a subclass to an object of a superclass. For example, we can upcast an instance of the `Circle` class to an instance of the `Shape` class. Once we have upcast the object, we can call the `draw()` method on the `Shape` object and the `draw()` method from the `Circle` class will be called.Here is an example of how we can use upcasting to improve the maintainability of our code:
public class Main { public static void main(String[] args) { Shape shape1 = new Circle(); Shape shape2 = new Square(); List shapes = new ArrayList<>(); shapes.add(shape1); shapes.add(shape2); for (Shape shape : shapes) { shape.draw(); } }}
In this example, we have created a list of shapes. We can add any type of shape to the list, and we can iterate over the list and call the `draw()` method on each shape. This code is much more maintainable than the previous example because we do not have to write separate code for each type of shape that we want to draw.
Upcasting is a powerful technique that can be used to improve the maintainability of your code. By upcasting, you can reduce the number of type-specific code that you need to write. This can make your code easier to read, understand, and maintain.
Frequently Asked Questions about "Get Up Cast"
This section addresses common questions and misconceptions surrounding the term "get up cast" in the context of programming and software development.
Question 1: What exactly is "get up cast" and how does it differ from "downcasting"?
Answer: "Get up cast," or upcasting, is a programming technique that converts a variable of a certain data type to a variable of a higher data type. This is often done to take advantage of the larger range or precision of the higher data type, or to allow the variable to be used in a context where the higher data type is required. In contrast, "downcasting" is the conversion of a variable of a higher data type to a variable of a lower data type, which involves potential data loss.
Question 2: When should upcasting be used and what are its potential benefits?
Answer: Upcasting is commonly used to improve code flexibility and performance. It allows objects of different classes to be treated as objects of a common superclass, enabling generic code that operates on various types. Additionally, upcasting can reduce the number of type conversions required, leading to improved performance and reduced code complexity.
Summary: Understanding the concept of "get up cast" is crucial for effective programming. Upcasting offers advantages in terms of code flexibility, performance optimization, and reduced complexity. However, it is essential to use upcasting judiciously, considering potential data loss and type safety concerns.
Conclusion on "Get Up Cast"
In summary, "get up cast," or upcasting, is a powerful programming technique that involves converting a variable of a certain data type to a variable of a higher data type. It offers several advantages, including increased flexibility, improved performance, and reduced code complexity.
Upcasting allows developers to write code that can operate on objects of different classes as if they were objects of a common superclass. This enhances code reusability and simplifies the handling of diverse data types. Moreover, upcasting can optimize performance by reducing the need for explicit type conversions, leading to faster execution times.
However, it is crucial to use upcasting judiciously, considering potential data loss and type safety concerns. Careful attention to data ranges and variable types is necessary to avoid errors and ensure the integrity of your code.
Overall, understanding and leveraging "get up cast" can significantly enhance the efficiency, flexibility, and maintainability of your software applications.
- Meet Amelie Warren Ed The Rising Star Of Hollywood
- Captivating Lee Yool A Guide To The Stars Life And Career
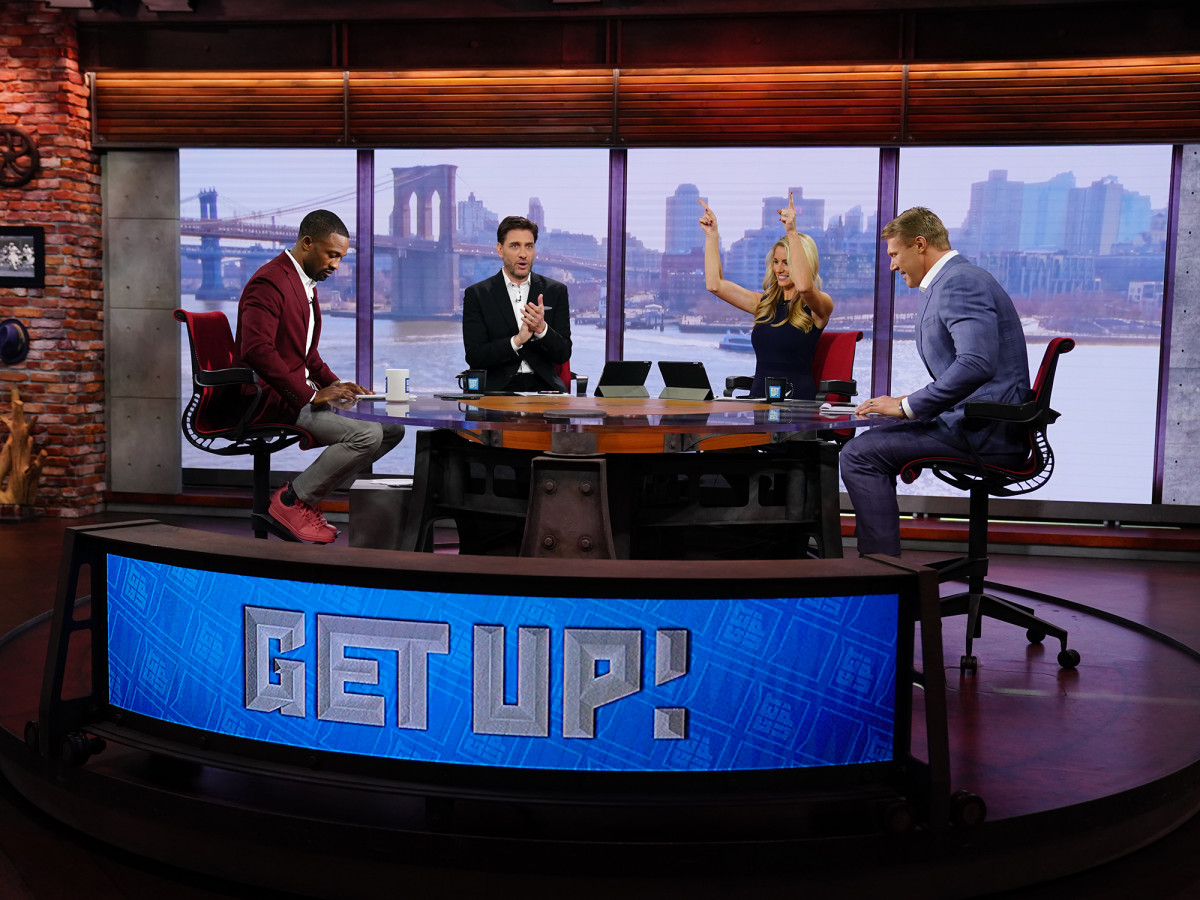
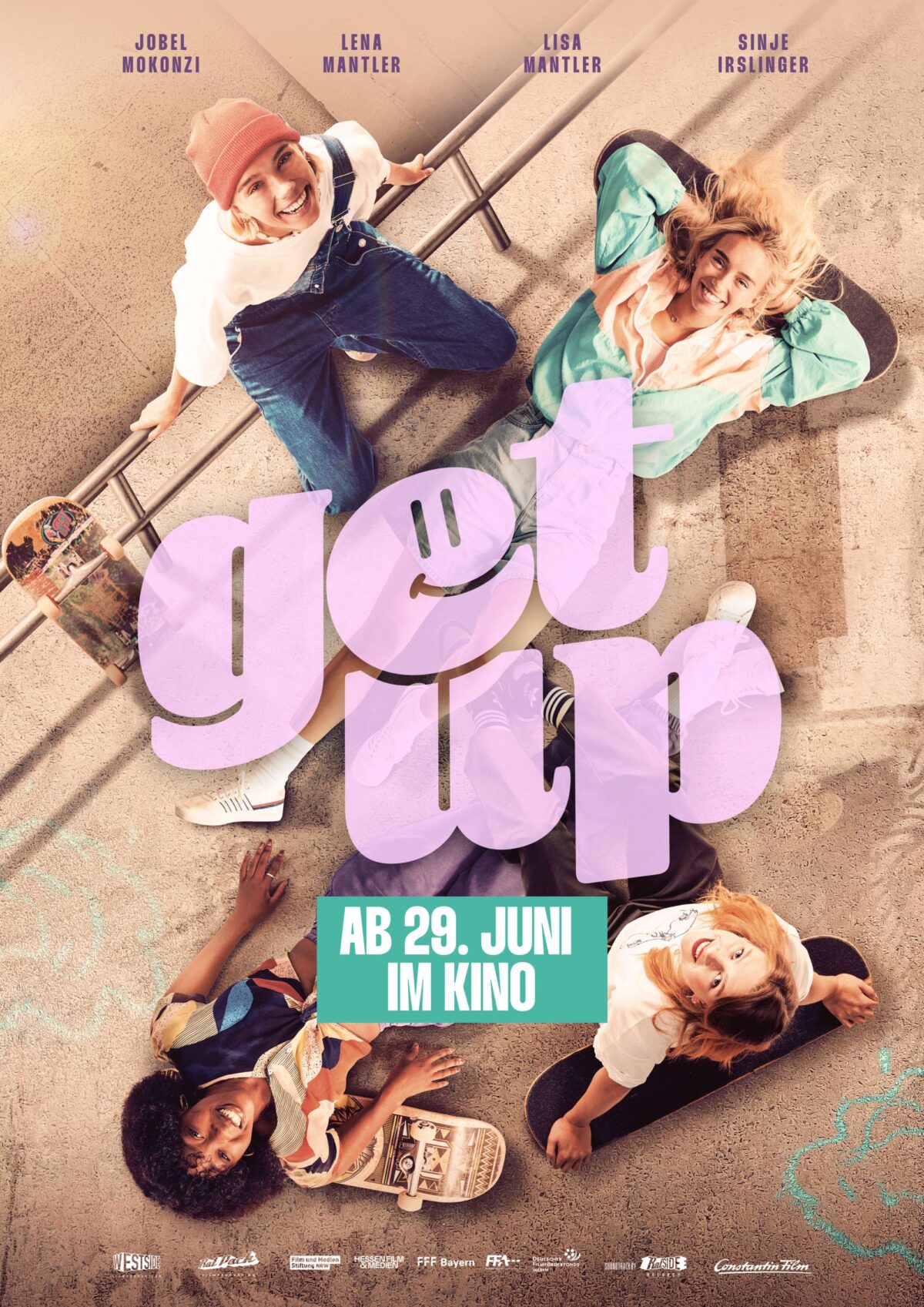
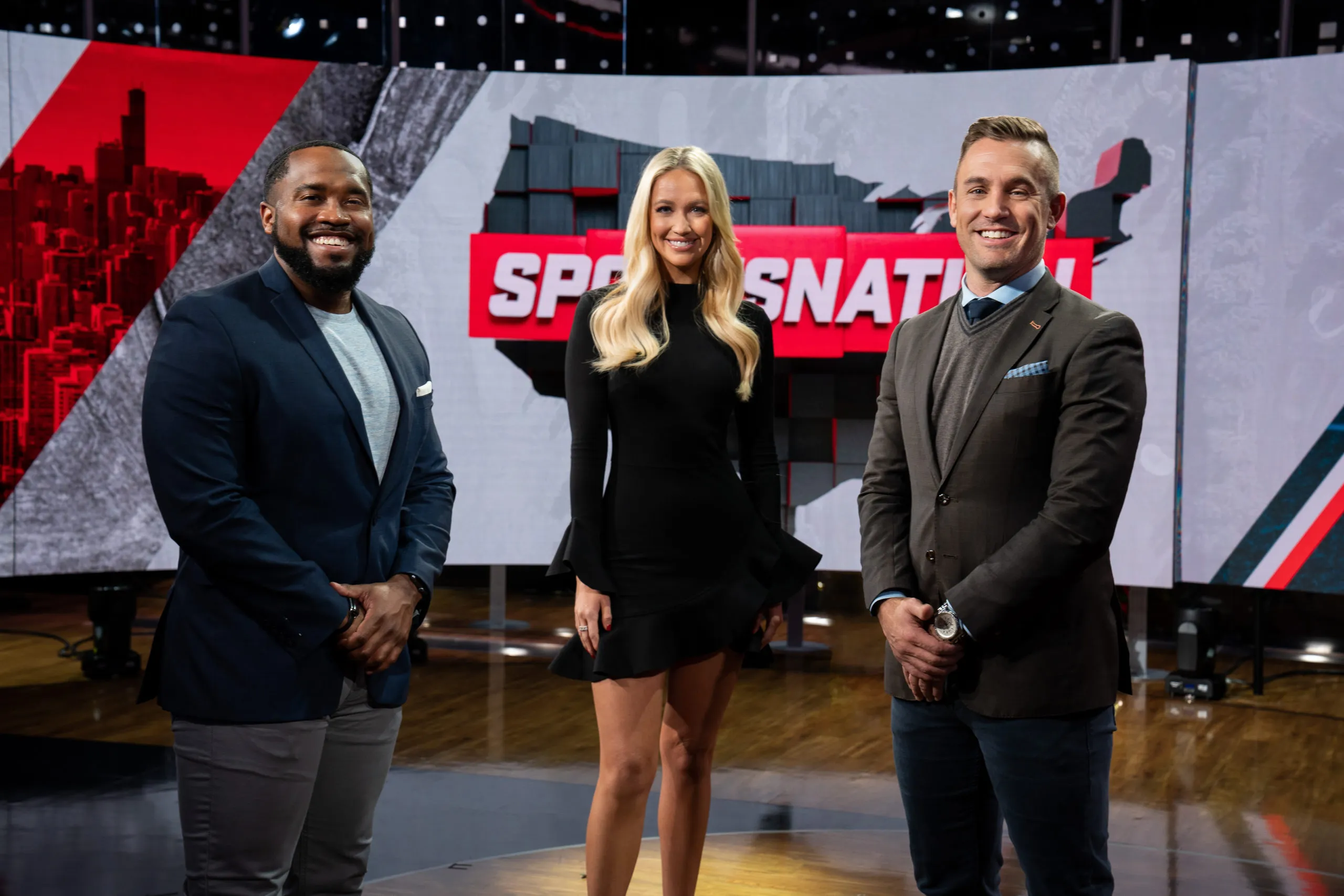